Effective Ways to Switch Branches in Git (2025)

Apply Now
```
This creates a new branch named `` and directly switches your working directory to that branch. Proper naming conventions, like using descriptive terms, help improve clarity.
```
This command effectively pivots your workspace to the specified branch, allowing you to work on it. However, if you have uncommitted changes, Git will prevent you from switching until you resolve those changes.
```
Using `git switch` provides clearer intent and reduces the chance of mistakes, making it a preferred method for many users. It is beneficial for beginners becoming accustomed to Git.
```
This incorporates all changes from the specified branch into your current branch, helping you integrate features seamlessly.
```
Using the `-d` option (short for delete) removes a branch safely, ensuring that it cannot be deleted if it has unmerged changes. For forced deletions, you can use `-D` instead.
```
This enables you to work on remote branches more efficiently, integrating team contributions smoothly into your local environment.
Effective Ways to Switch Branches in Git
Switching branches in Git is an essential skill for programmers and teams involved in software development. Branching allows developers to work on features, fixes, or experiments in isolation, ensuring that the main codebase remains stable. In this guide, we will explore effective techniques for switching branches in Git, optimizing your workflow for better productivity and collaboration. Understanding how to effectively switch branches is crucial for version control and effective branch management. Not only does it allow you to experiment freely with new features, but it also makes it easier to collaborate with other developers, resolve conflicts, and maintain a clean commit history. Throughout this article, we will cover various methods to switch branches, create new branches, and manage your branch workflow effectively using Git commands. Key Takeaways: * Efficient techniques for switching branches * Creating and managing branches * Merging and deleting branchesUnderstanding Git Branches and Command Basics
With multiple branches in a Git repository, it's important to understand the basics of branch management. Each branch represents a different line of development. By default, the main branch is often referred to as "main" or “master.” Let's delve into the essential commands and concepts related to viewing and managing branches.How to List Branches in Your Repository
To see which branches currently exist in your repository, you can use the command: ```bash git branch ``` This command will display a list of all local branches. The current branch you're on will be indicated with an asterisk (*). Moreover, to view both local and remote branches, you can utilize: ```bash git branch -a ``` This command allows you to identify branches that might have been created by collaborators on remote repositories.Verifying the Current Branch Status
Before operating on different branches, it’s wise to check which branch you’re working on. Use the command: ```bash git status ``` This command not only indicates the current branch, but also alerts you to any uncommitted changes. Utilizing this command regularly helps in maintaining clear context about your workspace.Creating New Branches for Development
When starting on a new feature or bug fix, creating a new branch is the first step. To create and switch to a new branch in one command, use: ```bash git checkout -bSwitching Between Branches Using Git
Switching branches is a straightforward process, but it may lead to conflicts if you have uncommitted changes. Let’s discuss how to change branches safely and efficiently.Using Git Checkout to Switch Branches
Traditionally, Git provides the `checkout` command to switch branches. To switch from your current branch to another branch: ```bash git checkoutIntroducing Git Switch Command
With the advent of Git version 2.23, a new command was introduced to simplify branch switching: `git switch`. This command enhances usability: ```bash git switchResolving Conflicts When Changing Branches
Occasionally, you may encounter conflicts when attempting to switch branches due to uncommitted changes. To resolve this, you can either commit those changes or stash them temporarily: ```bash git stash ``` Once you've stashed your changes, you can safely switch branches, and later pop the stash to restore your changes.Creating, Merging, and Deleting Branches Efficiently
Branch management in Git encompasses creating, merging, and deleting branches. Each of these operations are crucial for maintaining a clean and effective development process.How to Merge Branches
Once you've completed work on a feature branch, merging it back into the main branch is the next step. First, ensure you are on the branch you wish to merge into (typically the `main` branch): ```bash git checkout main ``` To merge the new feature branch, use: ```bash git mergeDeleting Unneeded Branches
Once a branch has served its purpose, you can clean up by deleting it: ```bash git branch -dTracking Remote Branches
In collaborative environments, you may need to track remote branches to collaborate effectively with your team. You can check the status of remote branches with: ```bash git fetch git branch -r ``` If you want to create a local branch that tracks a remote branch, use: ```bash git checkout --track origin/Maintaining a Smooth Git Workflow
To utilize Git effectively, it's essential to develop a strong workflow that integrates commands for managing branches, commits, and collaboration tools like GitHub.Best Practices for Git Branching
Follow a clear branching strategy, such as Gitflow or trunk-based development, which allows for improved collaboration. For instance, keeping feature branches short-lived encourages regularly integrating code and minimizes conflicts.Utilizing Git Commit Wisely
Frequent commits with clear messages improve the commit history. You can amend your last commit if necessary, using: ```bash git commit --amend ``` This feature is key to maintaining a tidy and understandable commit tree, helping others understand the progression of your project.Tracking Your Progress with Git Logs
Keep track of project changes and history by viewing commit logs: ```bash git log ``` This provides you with a comprehensive view of the commit history, including commit messages and authors, which can aid in debugging and understanding project evolution.Q&A: Common Git Branching Questions
What is the difference between Git checkout and Git switch?
Git checkout is a traditional command used for switching branches, while git switch is a newer command specifically designed for changing branches, making it easier and less error-prone.Can I merge a branch that has conflicts without resolving them?
No, Git prevents merging branches with unresolved conflicts. You must first resolve any conflicts before performing a merge operation.How do I view all branches, including remote branches?
To view both local and remote branches, use the command `git branch -a`.What happens to uncommitted changes when switching branches?
If there are uncommitted changes, Git will prevent you from switching branches until the changes are either committed or stashed.What strategies can I adopt for better branch management?
Using clear naming conventions, regularly merging feature branches into the main branch, and employing a collaborative branching strategy can significantly enhance branch management.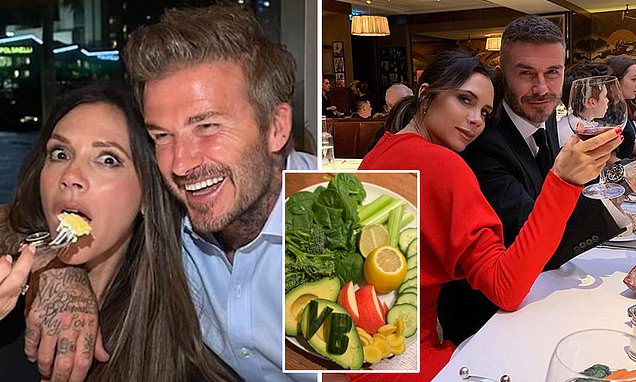
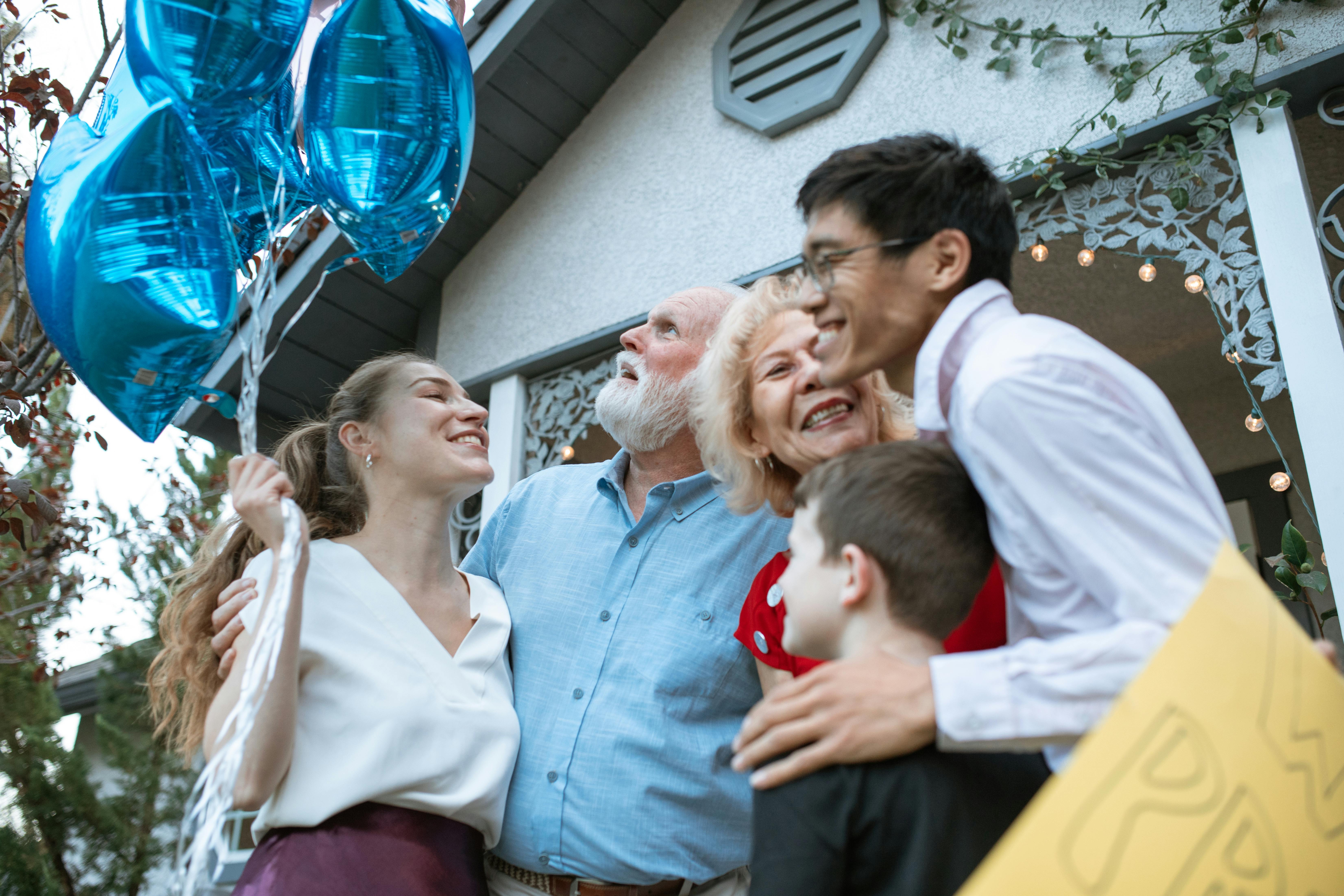